How to Download YouTube Videos using Python
How to Download YouTube Videos using Python
Send download link to:
YouTube is the biggest video streaming website in the world. All of us almost daily visit YouTube. You can find the videos on almost every topic in the world and not just entertainment. There are millions of creators on YouTube creating content daily which gives YouTube a unique advantage. You can find any kind of content you wish like cooking, adventure, science, tutorial for anything and everything. I have personally learned so many thing through YouTube weather it’s a new programming language, some machine learning problem or survival cooking.
Most of us want to download the videos that we like but there is no efficient way to do so if we have to download multiple videos. In this tutorial we will see how we can use Python to download YouTube Videos.
We will use a library specifically designed for YouTube called pytube3. This library makes it easy to download any video or a playlist from YouTube. You can read more about it here https://pypi.org/project/pytube3/. Be careful while using this library as some of the methods given in the documentation doesn’t work as expected. But using below script you can download any YouTube video. So let’s get into the code:
First install the library and import it:
pip install pytube3
from pytube import YouTube
Now we will go to this link https://www.youtube.com/watch?v=vLnPwxZdW4Y and downlod this video of C++ tutorial.
link = "https://www.youtube.com/watch?v=vLnPwxZdW4Y"
yt = YouTube(link)
stream = yt.streams.first()
stream.download()
Output:

You can find the downloaded video in your working directory.
Next, let’s explore how we would view what video streams (video quality) are available:
yt.streams.all()
Output:
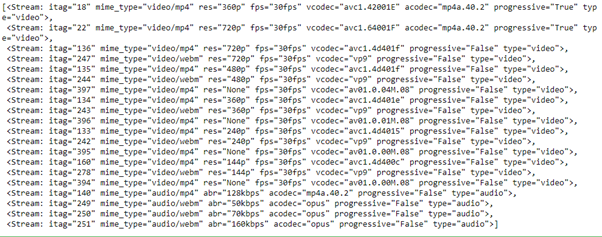
As you can see there are a lot of different video qualities available. You can download any of these by using there itag as below:
stream = yt.streams.get_by_itag('22')
stream.download()
You may notice that some streams listed have both a video codec and audio codec, while others have just video or just audio; this is a result of YouTube supporting a streaming technique called Dynamic Adaptive Streaming over HTTP (DASH).
In the context of pytube, the implications are for the highest quality streams; you now need to download both the audio and video tracks and then post-process them with software like FFmpeg to merge them.
The legacy streams that contain the audio and video in a single file (referred to as “progressive download”) are still available, but only for resolutions 720p and below.
To only view these progressive download streams:
yt.streams.filter(progressive=True).all()
Conversely, if you only want to see the DASH streams (also referred to as “adaptive”) you can do:
yt.streams.filter(adaptive=True).all()
You can also download a complete Youtube playlist:
#From pytube import Playlist
playlist = Playlist("https://www.youtube.com/watch?v=Edpy1szoG80&list=PL153hDY-y1E00uQtCVCVC8xJ25TYX8yPU")
#For video in playlist:
yt = YouTube(video)
stream = yt.streams.first()
stream.download()
That is all on YouTube downloads. Using above scripts you can download any video.