How to Use Google API in Python | Web scraping Python
How to Use Google API in Python | Web scraping Python
Send download link to:
Google provides API for almost all of its services like Maps, Location, Places, Gmail, Search, Translate etc. It has the most powerful set of API and are most widely used. Google Maps API is used almost on every app and webpage that uses maps or locations. Many of these API can be very easily used to get data from Google like Maps, Location, Places etc.
In this tutorial first we will see how to create Google API keys and later we will use it get directions between London and Paris.
Creating Google API keys
You must have at least one API key associated with your project.
To get an API key:
- Visit the Google Cloud Platform Console.
- Click the project drop-down and select or create the project for which you want to add an API key.
- Click the menu button and select APIs & Services > Credentials.
- On the Credentials page, click Create credentials > API key.
The API key created dialog displays your newly created API key. - Click Close.
The new API key is listed on the Credentials page under API keys.
(Remember to restrict the API key before using it in production.)
The first step is to create a new project. Once you’re logged into your Google account, visit the Google Cloud Platform page. If it’s your first time there may be terms you’ll need to accept, but you’ll eventually get to a blank looking page like this:
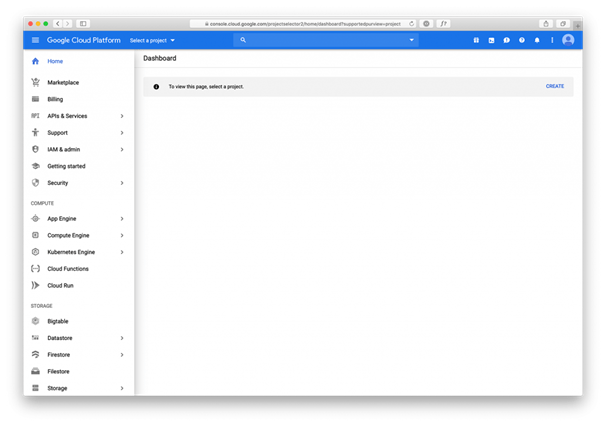
At this point, choose the Select a project dropdown and create a new project:
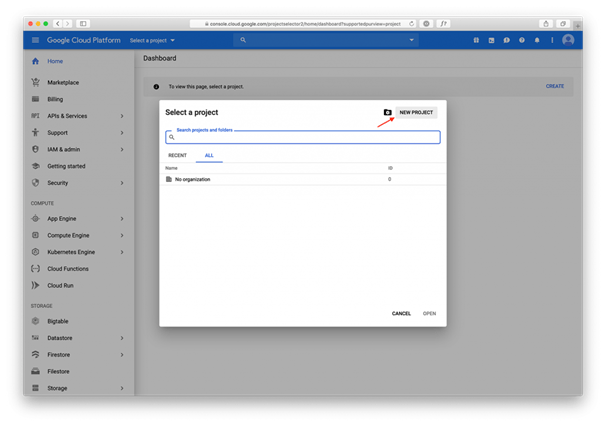
Give the project a name and click Create:
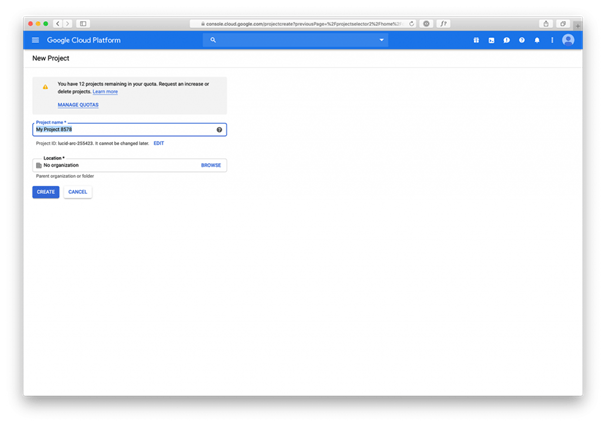
Once the new project is created, you’ll end up on the Google Cloud Platform dashboard.
The next step is to enable the APIs and Services you want. Find the APIs & Services link and click on the Dashboard:
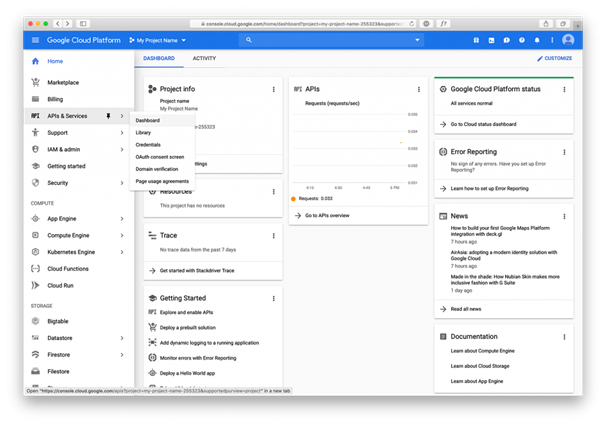
Once there, click on the “Enable APIs & Services” link:
This will take you to the wonderful world of Google APIs.
For this tutorial we will use Directions API so do enable that following above procedure.
After creating Keys and enabling API we need to add payment method for our project. Some Google APIs charge for usage after crossing the limit, and you need to enable billing before you can start using these APIs. If you enable billing for a project, then every billable API in that project is billed, based on the project’s usage.
Google Maps API provides you 200$ credits every month free of cost. If you are using more than 200$ per month then you have to pay as per the use.
To attach a billing account to a project follow below procedure:
- API is already created that mean you are already logged in to the account. If you are signout then go to the Google Cloud Platform console and sign in and select the project where you want to attach the billing account .
- Open the console left side menu and select Billing.
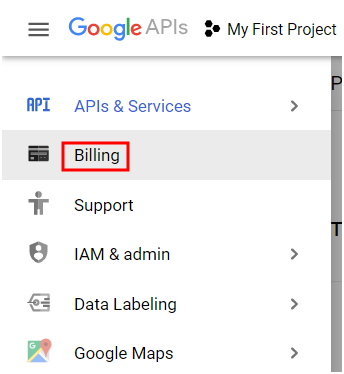
- Click the New billing account button.(note first you need to open the billing account list by clicking the name of your existing billing account near the top of the page, and then click Manage billing account).
- Enter the name of the billing account and enter your billing information. The option you see depend on your billing address.
- Click Submit and Enable billing.
With this you are ready to use Google API.
Getting Directions using Google API
We can get directions between two places using Google’s Directions API. To do that using Python we need to install googlemapslibrary.
To install the library do a pip install and then import:
pip install googlemaps
import googlemaps
Import configparser to read properties file and extract API key
To keep our keys safe we should never enter them directly in the code. Instead we will usingConfigparser which is module to read data from a file. To learn more about Configparser go here https://docs.python.org/3/library/configparser.html .
First save your keys in text file as below:
[Google]
googleapikey = *******your key********
Then use config parser to read from this file:
import configparser
config = configparser.RawConfigParser()
config.read(filenames = 'google.txt’)
print(config.sections())
apikey = config.get('Google',‘googleapikey’)
Now connect to your Google Map account using your API key as below:
gm = googlemaps.Client(key = apikey)
Now we will use googlemapsdiections() method to get the direction between London and Paris. See detail code and output below:
from datetime import datetime
now = datetime.now()
directions_result = gm.directions("London",
"Paris",
mode="transit",
departure_time=now)
directions_result
Output:
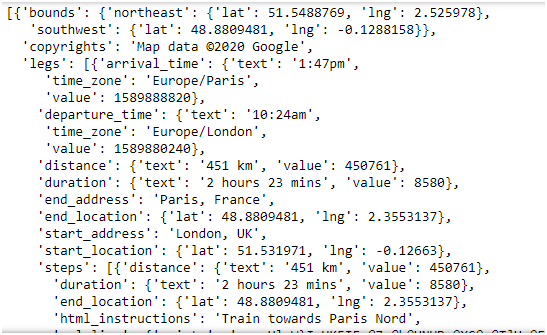
That’s all on Google API. Now Use Google API to save scraped data in to googlesheet